Creating a chatbot has become an engaging project for many aspiring developers and enthusiasts in the field of artificial intelligence. A chatbot is a computer program designed to simulate conversation with human users, particularly over the Internet. In this post, we will explore how to create a simple AI chatbot using Python, which is renowned for its ease of use and extensive libraries that simplify various programming tasks.
Building a chatbot can enhance user engagement, provide customer support, or even serve as an educational tool. With the increasing reliance on instant communication and automation, learning to create a simple AI chatbot can be a valuable skill. Python’s simplicity allows developers of all skill levels to jump into the world of AI without overwhelming complexity.
In this comprehensive guide, you will learn about key concepts such as Natural Language Processing (NLP) and Machine Learning (ML), as well as how to design user interactions that create an engaging experience. We’ll walk through a step-by-step process to create a simple AI chatbot, starting from setting up your development environment to running your program. You’ll also discover ways to enhance your chatbot with additional features, such as connecting to APIs for real-time information and using sentiment analysis to respond to users based on their emotional tone.
Whether you are a beginner looking to dip your toes into programming or an experienced developer aiming to explore AI, this guide provides all the information you need to create a simple AI chatbot in Python. By the end of this article, you will have the foundational knowledge and practical skills required to develop and enhance a chatbot tailored to your specific needs.
Step-by-Step Guide to Create a Simple AI Chatbot
Step 1: Setting Up Your Environment
Before we start coding, you need to set up your development environment. Ensure you have Python installed on your system. You can download it from Python’s official website.
Next, install the necessary libraries:
pip install nltk
pip install textblob
pip install requests
These libraries will help in various tasks, from natural language processing to making HTTP requests for API integration.
Step 2: Importing Required Libraries
Start by importing the required libraries. Here’s how you can set up your Python file:
import nltk
from nltk.chat.util import Chat, reflections
from textblob import TextBlob
import requests
The nltk.chat.util
module contains useful tools for building a chatbot, while TextBlob
will help in analyzing sentiments. The requests
library is essential for making API calls.
Step 3: Creating the Chatbot Pairs
In this step, you will define a set of predefined questions and responses to create a simple AI chatbot. The chatbot will match user inputs to these pairs and respond accordingly.
pairs = [
[
r"my name is (.*)",
["Hello %1, how can I assist you today?",]
],
[
r"hi|hey|hello",
["Hello!", "Hey there!",]
],
[
r"what is your name?",
["I am a chatbot created in Python.",]
],
[
r"how are you?",
["I'm just a bunch of code, but thanks for asking!",]
],
[
r"(.*) help (.*)",
["I can help you with programming questions!",]
],
[
r"quit",
["Thank you for chatting. Goodbye!",]
],
]
Each pair consists of a regex pattern and a corresponding response. The regex patterns allow for flexibility in user input, making your chatbot more interactive.
Step 4: Creating the Chatbot Function
Next, create a function that will initialize the chatbot with the defined pairs:
def chatbot():
print("Hello! I am your chatbot. Type 'quit' to exit.")
chat = Chat(pairs, reflections)
chat.converse()
The reflections
variable is a dictionary that helps the chatbot understand common phrases, making it more interactive and responsive to users.
Step 5: Running the Chatbot
Finally, you will run the chatbot function:
if __name__ == "__main__":
chatbot()
When you run this script, you will see a prompt where you can start chatting with your chatbot! This initial setup lays the groundwork for your journey to create a simple AI chatbot.
Enhancing Your Chatbot
Once you have the basic framework of your chatbot set up, it’s time to enhance its capabilities and make it more interactive.
Adding More Responses
To make your chatbot more versatile, consider adding additional responses based on user input. Here’s how to extend the pairs:
pairs.extend([
[
r"what can you do?",
["I can answer questions about programming, provide information, and assist with tech-related queries.",]
],
[
r"tell me a joke",
["Why do programmers prefer dark mode? Because light attracts bugs!",]
],
[
r"(.*) your favorite color?",
["As a chatbot, I don't have preferences, but blue is popular among many people!",]
],
])
By expanding the response pairs, you enhance your chatbot’s conversational abilities and engagement level, making it more enjoyable for users.
Incorporating Sentiment Analysis
You can also incorporate sentiment analysis to make your chatbot more responsive and engaging. Sentiment analysis can determine the user’s emotional state and tailor responses accordingly. To implement sentiment analysis, consider using the previously installed TextBlob library.
Here’s a simple implementation using TextBlob:
def analyze_sentiment(user_input):
analysis = TextBlob(user_input)
if analysis.sentiment.polarity > 0:
return "I'm glad you're feeling positive!"
elif analysis.sentiment.polarity < 0:
return "I'm sorry to hear that. Is there anything I can do to help?"
else:
return "It seems like you're feeling neutral."
You can call this function within your main chat loop to give feedback based on the user’s emotional tone. For example:
def chatbot():
print("Hello! I am your chatbot. Type 'quit' to exit.")
chat = Chat(pairs, reflections)
while True:
user_input = input("You: ")
if user_input.lower() == "quit":
print("Thank you for chatting. Goodbye!")
break
sentiment_response = analyze_sentiment(user_input)
print("Chatbot:", sentiment_response)
chat.respond(user_input)
Connecting to APIs
Integrating APIs can significantly enhance your chatbot’s functionality. You can connect to weather APIs, news APIs, or even your own custom APIs to provide real-time information.
For example, if you want your chatbot to provide weather updates, consider using the OpenWeatherMap API:
- Sign Up for an API Key: Create an account on OpenWeatherMap and obtain your API key.
- Use the Requests Library to Fetch Data:
pip install requests
- Integrate it into Your Chatbot:
def get_weather(city):
api_key = "your_api_key"
base_url = "http://api.openweathermap.org/data/2.5/weather?"
complete_url = f"{base_url}q={city}&appid={api_key}"
response = requests.get(complete_url)
data = response.json()
if data["cod"] != "404":
weather_description = data["weather"][0]["description"]
return f"The weather in {city} is currently {weather_description}."
else:
return "City not found."
Add this function to your response pairs to handle weather queries:
pairs.append([
r"weather in (.*)",
[get_weather,]
])
By connecting your chatbot to external APIs, you provide users with up-to-date information, making their experience richer and more informative.
Testing and Deployment
Once development is completed to create a simple AI chatbot, it’s crucial to test its functionality. Create a list of potential user questions and see how well your chatbot handles them. Based on the feedback, refine your responses and improve the conversation flow.
Creating Test Scenarios
Consider different scenarios to test your chatbot effectively:
- Normal Conversations: Engage the chatbot in standard dialogue to assess how it handles everyday interactions.
- Edge Cases: Test unusual phrases or unexpected inputs to evaluate its robustness.
- Sentiment Reactions: Deliberately use positive, negative, and neutral statements to ensure that the sentiment analysis works as intended.
Deployment Options
When you’re satisfied with the performance, consider deploying your chatbot. You can host it on platforms like Heroku or AWS for broader accessibility. Additionally, integrating your chatbot into messaging platforms like Facebook Messenger or Slack can expand its usability.
- Heroku Deployment: Heroku allows for easy deployment of Python applications.
- AWS Deployment: If you prefer AWS, you can deploy your chatbot on an EC2 instance. AWS provides scalability and flexibility for your applications.
- Messaging Platforms: Utilize APIs from platforms like Facebook or Slack to connect your chatbot and provide a seamless user experience.
Troubleshooting Common Issues
As you work on your chatbot, you might encounter some common issues. Here are some tips for troubleshooting:
1. Regex Patterns Not Matching
If the chatbot doesn’t respond as expected, double-check your regex patterns. Ensure they correctly match the expected user inputs. Test various variations of user inputs to confirm that your patterns are robust.
2. Limited Responses
If your chatbot feels repetitive, consider expanding the pairs and adding more diverse responses. A richer set of responses can make conversations more engaging and dynamic.
3. Connection Issues with APIs
Ensure that your API key is valid and that you’re correctly handling API responses. If your chatbot fails to retrieve data from an API, check the documentation for any changes in the API structure or requirements.
4. Performance Optimization
If your chatbot becomes slow or unresponsive, investigate potential performance bottlenecks. Optimize your code by minimizing API calls or using efficient data structures.
Exploring Advanced Features
As you become more comfortable with the basics to create a simple AI chatbot, consider integrating advanced features to further enhance its capabilities.
1. Machine Learning Integration
Incorporate machine learning techniques to improve the chatbot’s ability to understand and respond to user inputs. Libraries like scikit-learn
and TensorFlow
can be useful for building more sophisticated models.
- Training Data: Gather a diverse set of user inputs and responses to train your model effectively.
- Model Evaluation: Regularly evaluate the model’s performance and make adjustments as necessary to improve accuracy.
2. Multi-Language Support
If you want to reach a broader audience, consider implementing multi-language support in your chatbot. Use libraries like googletrans
for language translation or develop separate response pairs for different languages.
3. Personalization Features
To create a more tailored experience for users, consider adding personalization features. For example, store user preferences or past interactions, allowing the chatbot to remember users and tailor conversations accordingly.
user_preferences = {}
def store_user_preference(user_id, preference):
user_preferences[user_id] = preference
def get_user_preference(user_id):
return user_preferences.get(user_id, None)
4. Visual Interfaces
Enhance user engagement by creating a visual interface for your chatbot. Use frameworks like Flask or Django to develop a web-based chatbot, or utilize GUI libraries like Tkinter for desktop applications.
5. Analytics and Feedback Collection
Integrate analytics tools to track user interactions and gather feedback. This data can provide insights into user behavior and preferences, helping you to improve your chatbot continuously.
Conclusion: The Journey to Create a Simple AI Chatbot
Creating a simple AI chatbot using Python is a fantastic way to dive into the world of artificial intelligence and natural language processing. By following the steps outlined in this guide, you have developed a basic chatbot that can engage users in conversation.
As you continue to learn and explore, consider integrating more advanced features such as machine learning for improved responses, connecting your chatbot to external APIs, or even deploying it on a messaging platform.
For further reading and resources on AI and chatbot development, visit Towards Data Science or Analytics Vidhya. Additionally, resources like Chatbots Magazine and The Bot Platform offer insights into chatbot strategies and technologies that can help you enhance your project.
With the skills you’ve gained here, you are well on your way to creating not just a chatbot but an engaging digital companion that can assist users and provide valuable interactions. Keep experimenting and expanding your knowledge, and you’ll find countless opportunities in the field of artificial intelligence.
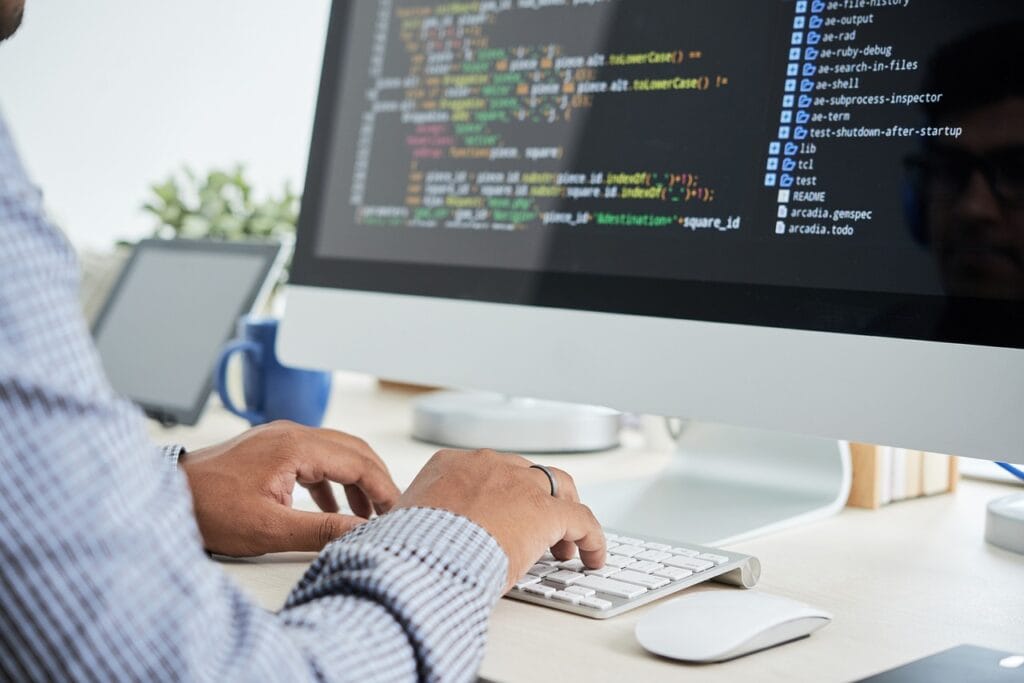